Retrieval-Augmented Generation
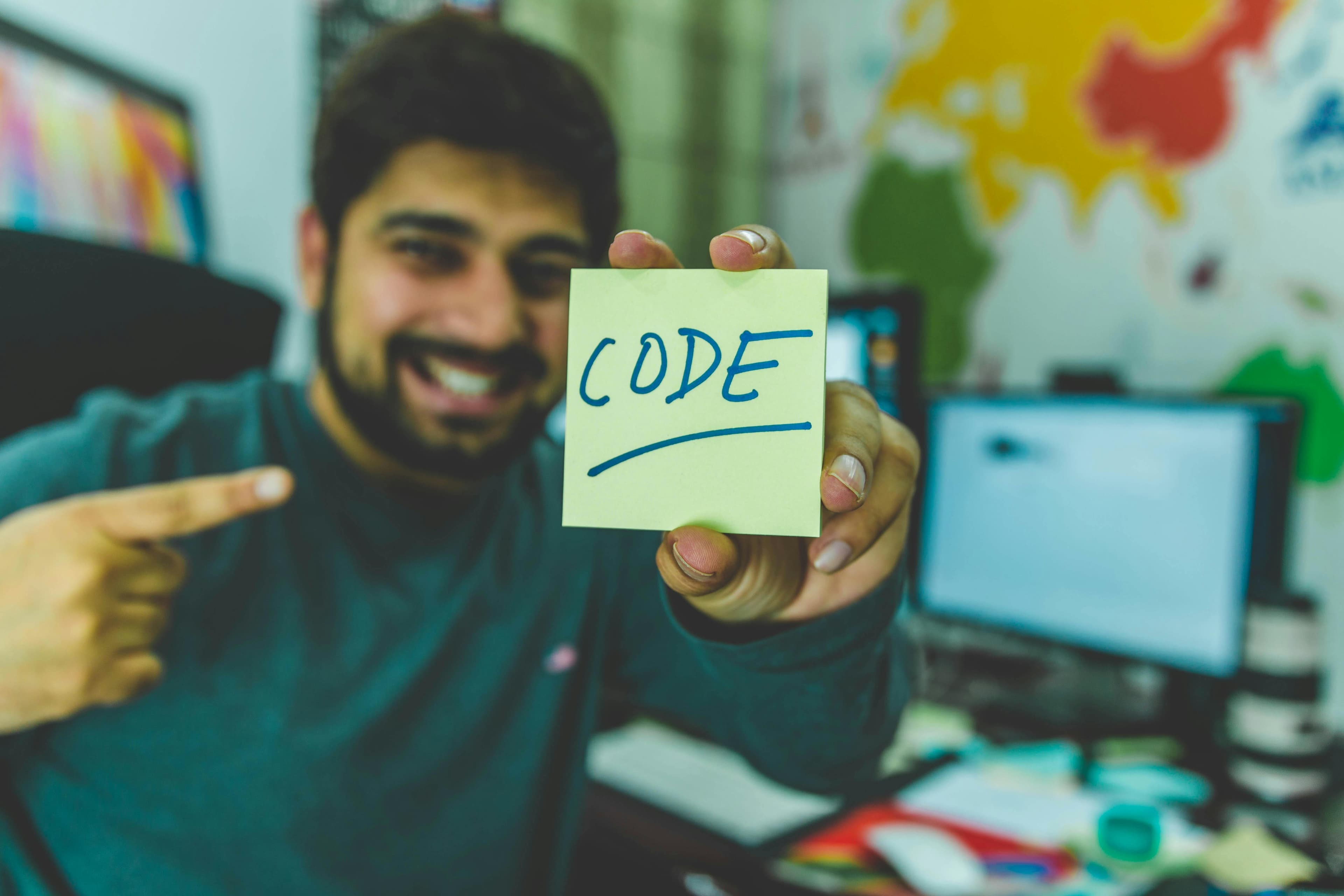
Understanding RAG (Retrieval-Augmented Generation) in Code
AI, with large language models (LLMs)
Use Retrieval-Augmented Generation (RAG)
To obtain new DATA to update the LLM previously trained data:
Retrieves: It pulls relevant information from external data sources (like databases or documents). Augments: It then feeds this retrieved information to an LLM. Generates: This allows the LLM to produce more accurate and contextually relevant responses. Essentially, RAG helps LLMs overcome their limitations of having a "knowledge cutoff" by providing them with up-to-date and specific information.
This Python script implements a Retrieval-Augmented Generation (RAG) pipeline using OpenAI's API and Ollama for embeddings and chat completion. RAG is an approach where a model retrieves relevant context from an external knowledge base before generating a response. Let’s break down the key components:
- What is RAG? Retrieval-Augmented Generation (RAG) is an AI framework where:
Retrieve: It fetches the most relevant information from a database (vault.txt in this case). Augment: It appends this relevant information to the user query. Generate: It sends the augmented query to a language model to produce a final response. This is useful because LLMs (Large Language Models) have knowledge limits—RAG helps by providing external knowledge dynamically.
- Key Components of This Code A. Retrieving Relevant Context
def get_relevant_context(rewritten_input, vault_embeddings, vault_content, top_k=3):
This function finds the most relevant pieces of text from vault.txt using cosine similarity between:
- The query's embedding (rewritten_input).
- The precomputed embeddings of the vault_content.
- top_k=3 controls how many top relevant results are used.
B. K Parameter - What is top_k?
top_k = min(top_k, len(cos_scores))
top_indices = torch.topk(cos_scores, k=top_k)[1].tolist()
-
top_k selects the top K most relevant pieces of retrieved text.
-
It ensures the model gets only the most important information rather than being overloaded. Why is K important?
-
Higher K (e.g., top_k=5) → More context is retrieved, improving accuracy but increasing computational load.
-
Lower K (e.g., top_k=1) → Less context, faster response, but potentially missing key details.
C. Query Rewriting (rewrite_query)
def rewrite_query(user_input_json, conversation_history, ollama_model):
- Instead of directly using the user’s query, it rephrases it using the past conversation.
- This is important because LLMs respond better to well-structured queries.
- Uses OpenAI API (client.chat.completions.create) to generate a better query.
D. Augmenting the User Query
if relevant_context:
user_input_with_context = user_input + "\n\nRelevant Context:\n" + context_str
- If relevant documents are found, they are appended to the user query before sending it to the model.
- This ensures the LLM has both the user’s intent and relevant data.
E. Generating a Response
response = client.chat.completions.create(
model=ollama_model,
messages=messages,
max_tokens=2000,
)
- The final query (with context) is sent to OpenAI’s API for response generation.
-
Why This Code Uses RAG
- Improves accuracy by giving the AI external data.
- Handles knowledge gaps in the base LLM.
- Ensures relevance by fetching only important information from vault.txt instead of the entire document.
-
Summary
Concept | Explanation |
---|---|
Retrieval-Augmented Generation | Enhances LLMs by retrieving relevant context before generating responses. |
Top_k | Controls the number of retrieved results (higher k = more context). |
Embeddings | Used to find the most relevant text in vault.txt using cosine similarity. |
Query Rewriting | Ensures queries are well-structured for better responses. |
Final Response | The query + retrieved context is sent to OpenAI’s API for response generation. |
Postgres Rag
To set up PostgreSQL for both development and production with Docker in a professional, cloud-ready manner, it's crucial to establish best practices that emphasize security, configuration management, scalability, and separation of environments. Here’s a precise and clear plan for this setup:
1. Define Separate Environments
- Development Environment: Local Docker container with simplified configuration, often with fewer restrictions for ease of use.
- Production Environment: Cloud-hosted instance with stricter security settings, backups, and monitoring.
2. Configuration Management Using Environment Variables
-
Use environment variables to manage PostgreSQL configurations for both development and production. Avoid hardcoding sensitive information (like passwords).
-
Environment-Specific Files: Use separate
.env
files for each environment:.env.development
: Holds environment variables for local development..env.production
: Holds environment variables for production.
-
In your Docker setup, you can load these files as needed by the environment:
bash docker run --env-file .env.development -d postgres
3. Database Credentials and Security
- Development: It’s common to use a default username (like
postgres
) and password for local development to streamline setup. However, for security and consistency:- Use
.env.development
to store credentials. - Ensure that even in development, password access is used instead of relying on trusted local connections.
- Use
- Production:
- Use a Secure Password: Generate a strong, unique password.
- Environment Secrets: Store passwords securely using a secrets manager (such as AWS Secrets Manager, Azure Key Vault, or Docker Secrets in Swarm mode).
- Least Privilege: Create a specific database user with only the necessary privileges for your application, avoiding the
root
user whenever possible.
4. Docker Compose for Simplified Development Setup
-
Using Docker Compose can streamline managing PostgreSQL and your application’s containers locally.
-
Example
docker-compose.yml
for local development:yaml version: '3' services: db: image: postgres:latest container_name: myapp_db environment: POSTGRES_USER: ${POSTGRES_USER} POSTGRES_PASSWORD: ${POSTGRES_PASSWORD} POSTGRES_DB: ${POSTGRES_DB} ports: - "5432:5432" volumes: - db_data:/var/lib/postgresql/data volumes: db_data:
-
Add environment variables to your
.env.development
file:env POSTGRES_USER=root POSTGRES_PASSWORD=your_dev_password POSTGRES_DB=myapp_dev
5. Data Persistence and Backups
- Development: Use Docker volumes to persist data locally across container restarts.
- Production:
- Cloud Provider Storage: Mount cloud storage volumes for database persistence.
- Automated Backups: Set up scheduled backups using cloud-native services or database backup tools (e.g.,
pg_dump
).
6. Networking and Access Control
- Development:
- Expose PostgreSQL on a local port (e.g.,
5432:5432
) only when necessary. Limit access to local use.
- Expose PostgreSQL on a local port (e.g.,
- Production:
- Use a private network to restrict direct external access to the PostgreSQL instance.
- Only allow your application services to communicate with the PostgreSQL instance.
- If public access is necessary (e.g., for administration), restrict it via IP whitelisting and strong firewalls.
7. Database Migrations and Seeding
- Development: Use a tool like Flyway or Alembic to handle migrations for development databases. Seed data if needed.
- Production: Carefully manage migrations to avoid disrupting live data, ideally with automated CI/CD workflows that handle migrations with minimal downtime.
8. Monitoring and Logging
- Development: Basic logging is typically sufficient.
- Production:
- Logging: Enable PostgreSQL logging to capture queries, errors, and performance metrics. Consider using tools like Prometheus and Grafana for monitoring.
- Alerting: Set up alerts for critical metrics (e.g., high CPU, memory usage, slow queries).
9. Sample Workflow with Docker Compose for Both Environments
For Development:
-
Use
docker-compose.yml
as shown above. -
Run with the command:
bash docker-compose --env-file .env.development up -d
For Production (Example Docker Compose File):
yaml
version: '3'
services:
db:
image: postgres:latest
environment:
POSTGRES_USER: ${POSTGRES_USER}
POSTGRES_PASSWORD: ${POSTGRES_PASSWORD}
POSTGRES_DB: ${POSTGRES_DB}
volumes:
- db_data:/var/lib/postgresql/data
networks:
- internal_network
volumes:
db_data:
networks:
internal_network:
driver: bridge
In production, configure .env.production
securely and use orchestration (e.g., Docker Swarm or Kubernetes) with dedicated cloud secrets management to handle credentials.
Summary Checklist for Professional, Cloud-Ready PostgreSQL with Docker
- Separate
.env
files per environment, no hardcoded secrets. - Docker Compose for development; secure Docker configurations for production.
- Use cloud services for persistence and automated backups.
- Apply strict access controls for production.
- Implement database migration tools and CI/CD workflows.
- Enable monitoring, logging, and alerting for production performance.
This approach ensures a secure, scalable, and maintainable PostgreSQL setup for both development and production.
Suggestions for Next Steps:a. Configure CI/CD pipeline to automate migration and deployment.
b. Add Docker health checks to ensure container readiness before running dependent services.
You said:
Be very clear , I have apple mac mini m1 and desktop version postgres , I will be using llama 3.2 as chat RAG model , what priveledges do I require , think long hard be concise be professional
ChatGPT said:
To run PostgreSQL on an Apple Mac Mini M1, manage it securely for a development environment, and support a Llama 3.2 RAG (Retrieval-Augmented Generation) model setup, you should carefully configure user privileges, permissions, and access controls.
1. PostgreSQL Role and Privilege Configuration
For Development (Mac Mini M1)
- PostgreSQL Admin User: Avoid using the root or
superuser
role for daily development to reduce security risks. - Primary Application User: Create a dedicated PostgreSQL user for your RAG model’s database interactions. This user should have only the minimum required privileges to perform data operations (e.g.,
SELECT
,INSERT
,UPDATE
). - Role Example:
-
Application Role (
rag_user
): Grants only read/write access to relevant tables, not admin privileges.sql CREATE USER rag_user WITH PASSWORD 'your_secure_password'; GRANT CONNECT ON DATABASE my_database TO rag_user; GRANT USAGE ON SCHEMA public TO rag_user; GRANT SELECT, INSERT, UPDATE ON ALL TABLES IN SCHEMA public TO rag_user; ALTER DEFAULT PRIVILEGES IN SCHEMA public GRANT SELECT, INSERT, UPDATE ON TABLES TO rag_user;
-
- Superuser Access (Rarely Used): Reserve
superuser
or root access for database setup, maintenance, or schema changes, and avoid using it in regular operations.
2. Secure Database Access
- Local Access Only for Development: Keep PostgreSQL running on
localhost
without exposing it to external networks to reduce security risks. This limits access to your Mac Mini only, blocking external requests unless explicitly allowed. - Strong Passwords: Use a strong password for each role, especially for any elevated privileges.
3. Environment-Specific Configuration Files
- Use separate environment configuration files (e.g.,
.env.development
) to manage credentials and prevent hardcoded passwords in your codebase. Load these variables dynamically in your RAG model environment to keep secrets out of code.
4. Database Security Practices
-
Disable Remote Connections (for Development): In
postgresql.conf
, configure PostgreSQL to accept only local connections by setting:conf listen_addresses = 'localhost'
-
Limit Roles: Avoid assigning unnecessary roles to your application user (like
CREATE
orDELETE
) unless explicitly needed by the RAG model.
5. Backup and Restore Privileges
-
Backup Role: Create a dedicated
backup_user
with limited permissions for database dumps, if needed. This allows secure, read-only access for backups.sql CREATE ROLE backup_user WITH LOGIN PASSWORD 'secure_password'; GRANT CONNECT ON DATABASE my_database TO backup_user; GRANT USAGE ON SCHEMA public TO backup_user; GRANT SELECT ON ALL TABLES IN SCHEMA public TO backup_user;
Summary Checklist for Professional Local Development Setup on Mac Mini M1
- Minimal Privileges: Use a dedicated
rag_user
with only required read/write privileges for your Llama 3.2 RAG model. - Localhost Access Only: Restrict PostgreSQL to local connections (
localhost
) to avoid unintended external access. - Environment Files: Store credentials in environment files (
.env.development
) to keep secrets out of code. - Separate Backup Role (Optional): Use a
backup_user
for read-only backup access, separate from application access.
These steps ensure a secure, professional-grade PostgreSQL configuration for local development with the Llama RAG model on your Mac Mini M1. This approach is also portable to production environments with only minor adjustments.
Suggestions for Next Steps:a. Configure automated environment variable loading for local and cloud development.
b. Test user role permissions by performing model-driven queries to ensure only necessary privileges are active.