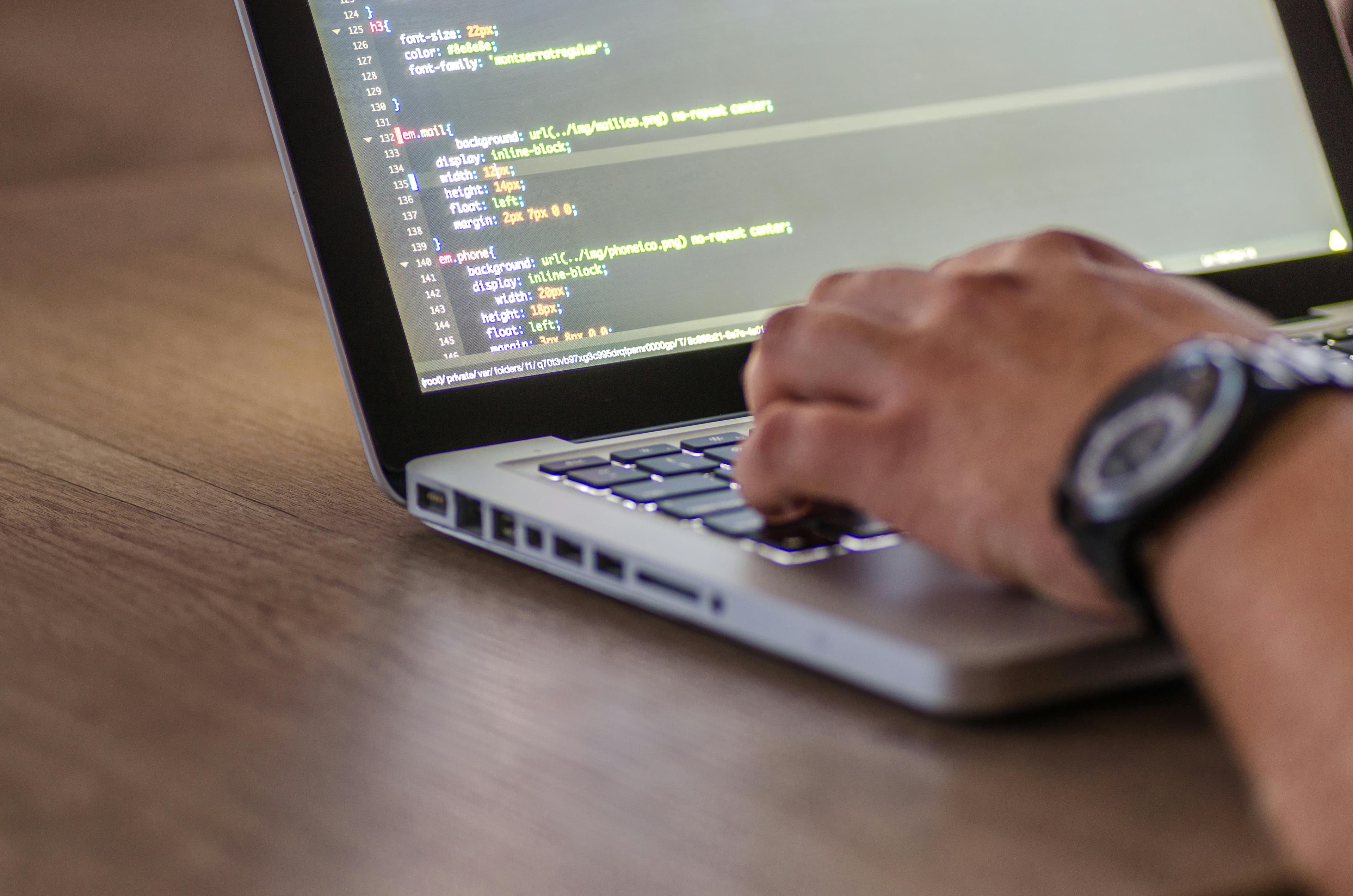
Machine-Learning-a-beginners-roadmap
Fundamentals of Deep Learning
-
What is Deep Learning?
A subset of ML using neural networks with multiple layers.
Neural Networks:
-
Structure: Input layer → Hidden layers → Output layer.
-
Activation Functions: Sigmoid, ReLU, Softmax, etc.
-
Backpropagation: Updating weights using gradient descent.
Popular Architectures:
Feedforward Neural Networks (FNN): Basic architecture.
Convolutional Neural Networks (CNN): For image data.
Recurrent Neural Networks (RNN): For sequential data like time series or text.
Intro Code
Machine learning (ML) has become one of the most sought-after fields in technology, driving innovations in artificial intelligence, automation, and data science. For beginners, the journey into ML can feel overwhelming due to the breadth of concepts involved. This roadmap provides a step-by-step guide, starting from foundational programming skills to fundamental ML models like Linear Regression.
1. Understanding the Foundations of Machine Learning
Before diving into ML algorithms, it's essential to have a strong foundation in software development and mathematical concepts.
Programming Prerequisites
Python: The most popular ML language, with libraries like NumPy, Pandas, and Scikit-learn.
Data Structures & Algorithms: Understanding lists, dictionaries, loops, and recursion is essential.
Version Control (Git): Keeping track of your projects efficiently.
Mathematics for Machine Learning
Linear Algebra: Vectors, matrices, and transformations.
Statistics & Probability: Mean, variance, distributions, and Bayes' theorem.
Calculus: Derivatives, gradients, and optimization techniques.
Getting Comfortable with Data
Data is the backbone of ML. Beginners should focus on how to collect, clean, and preprocess data before feeding it into models.
2. Introduction to Pandas & NumPy
What is Pandas?
Pandas is a Python library used for data manipulation and analysis. It provides powerful tools to:
-
Load and clean datasets
-
Perform data transformations
-
Handle missing values
Basic Pandas Operations:
import pandas as pd
# Load data
data = pd.read_csv('dataset.csv')
# View first few rows
data.head()
What is NumPy?
NumPy is a fundamental library for numerical computing, offering support for arrays and mathematical functions.
Basic NumPy Operations:
import numpy as np
# Create an array
array = np.array([1, 2, 3, 4, 5])
# Compute mean
print(np.mean(array))
3. Basics of Machine Learning: Supervised vs. Unsupervised Learning
Supervised Learning
Definition: Models learn from labeled data.
Examples: Linear Regression, Decision Trees, Neural Networks.
Unsupervised Learning
Definition: Models identify patterns in unlabeled data.
Examples: Clustering (K-Means), Dimensionality Reduction (PCA).
4. Introduction to Linear Regression
Linear regression is one of the simplest and most widely used ML algorithms.
Concept of Linear Regression
It predicts a continuous value based on input features.
Uses the equation Y = mX + b where:
Y = Dependent variable (output)
X = Independent variable (input)
m = Slope
b = Intercept
Example: Predicting House Prices
from sklearn.linear_model import LinearRegression import numpy as np
Sample data
X = np.array([1, 2, 3, 4, 5]).reshape(-1, 1) # Features
y = np.array([100, 200, 300, 400, 500]) # Target
# Train model
model = LinearRegression()
model.fit(X, y)
# Make prediction
prediction = model.predict([[6]])
print(prediction)
5. Roadmap for Beginners: Where to Go Next?
- Step 1: Learn Python & Essential Libraries
Master Python basics and practice using Pandas, NumPy, and Matplotlib.
- Step 2: Strengthen Your Math Skills
Focus on linear algebra, probability, and calculus for ML models.
- Step 3: Work on Small ML Projects
Predicting house prices using Linear Regression.
Sentiment analysis using Natural Language Processing (NLP).
- Step 4: Learn More Complex Models
Logistic Regression, Decision Trees, and Neural Networks.
Explore deep learning frameworks like TensorFlow and PyTorch.
- Step 5: Participate in Kaggle Competitions
Kaggle offers datasets and challenges to enhance practical ML skills.
-
What is ML?
The science of making computers learn patterns from data without being explicitly programmed.
-
Types of ML:
Supervised Learning: Learning from labeled data (e.g., regression, classification).
Unsupervised Learning: Finding patterns in unlabeled data (e.g., clustering, dimensionality reduction).
Reinforcement Learning: Learning through rewards and penalties.
-
Key Concepts:
Training, Validation, Testing: Splitting data for model evaluation.
- Overfitting/Underfitting: The balance between memorizing and generalizing.
- Bias-Variance Tradeoff: The balance between simplicity and complexity.
- Features and Labels: Input variables and outputs to predict.
- Gradient Descent: Algorithm to minimize a loss function.
- Evaluation Metrics: Accuracy, precision, recall, F1-score, etc.
Introduction to NLP (Natural Language Processing)
Core Concepts:
-
Tokenization: Breaking text into smaller units (e.g., words or subwords).
-
Embeddings: Mapping words to vector spaces (e.g., Word2Vec, GloVe).
-
Sequence-to-Sequence Models: For tasks like translation or summarization.
Key NLP Tasks:
-
Text Classification: Sentiment analysis, spam detection.
-
Named Entity Recognition (NER): Identifying entities (e.g., names, locations).
-
Language Modeling: Predicting the next word in a sequence.
-
Text Generation: GPT-like capabilities.
Advanced Topics in Neural Networks
Attention Mechanisms:
-
What it solves: Helps models focus on important parts of the input sequence.
-
Applications: Translation, summarization.
Transformers:
-
Replaces RNNs for NLP tasks.
-
Core idea: Self-attention + positional encoding.
-
Example: The architecture behind models like BERT and GPT.
Transfer Learning:
-
Reusing pre-trained models on a new task.
-
Popular models: BERT, GPT-3/4, T5.
LLMs (Large Language Models)
What Are LLMs?
- Models trained on massive text datasets to generate coherent, context-aware text.
Key Techniques:
-
Pre-training: Learning general language patterns.
-
Fine-tuning: Adapting the model for specific tasks.
Popular LLMs:
- GPT series, BERT, T5, LLaMA, etc.
Applications:
- Chatbots, text summarization, content creation, coding assistance.
Applied Topics in NLP and LLMs
Prompt Engineering:
-
Crafting inputs to guide LLM behavior effectively.
-
Zero-shot and Few-shot Learning:
-
Performing tasks with little to no task-specific training data.
Ethics in NLP:
-
Bias detection and mitigation.
-
Data privacy considerations.
Fine-Tuning vs. Inference APIs:
- Building custom models or leveraging cloud-hosted APIs.
Scaling and Optimization
-
Distributed Training: For handling large-scale models.
-
Model Compression: Quantization, pruning, and distillation.
-
Infrastructure: GPUs, TPUs, and cloud platforms.
Next Steps
-
Start Coding: Use Python libraries like TensorFlow, PyTorch, and Hugging Face Transformers.
-
Work on Datasets: Explore benchmarks like IMDB, Common Crawl, or SQuAD.
-
Build Projects: Start with text classification or summarization.
A cute, pixel art style
with clear facial features standing on a
. This depicted in colors. The scene is low-resolution and has flat, even light. The background should contain pixelated shapes.
Conclusion
Machine learning can seem overwhelming at first, but breaking it down into structured learning steps makes the journey easier. By focusing on programming, math, and data handling before diving into ML models, beginners can develop a solid foundation for long-term success.
Would you like to explore any specific ML topics in greater detail? 🚀